- <RelativeLayout xmlns:androclass="http://schemas.android.com/apk/res/android"
- xmlns:tools="http://schemas.android.com/tools"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- android:paddingBottom="@dimen/activity_vertical_margin"
- android:paddingLeft="@dimen/activity_horizontal_margin"
- android:paddingRight="@dimen/activity_horizontal_margin"
- android:paddingTop="@dimen/activity_vertical_margin"
- tools:context=".MainActivity" >
- <TextView
- android:id="@+id/textView1"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_alignParentTop="true"
- android:layout_marginTop="30dp"
- android:text="Audio Controller" />
- <Button
- android:id="@+id/button1"
- style="?android:attr/buttonStyleSmall"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_alignLeft="@+id/textView1"
- android:layout_below="@+id/textView1"
- android:layout_marginTop="48dp"
- android:text="start" />
- <Button
- android:id="@+id/button2"
- style="?android:attr/buttonStyleSmall"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_alignTop="@+id/button1"
- android:layout_toRightOf="@+id/button1"
- android:text="pause" />
- <Button
- android:id="@+id/button3"
- style="?android:attr/buttonStyleSmall"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_alignTop="@+id/button2"
- android:layout_toRightOf="@+id/button2"
- android:text="stop" />
- </RelativeLayout>
- package com.example.audioplay;
- import android.media.MediaPlayer;
- import android.os.Bundle;
- import android.os.Environment;
- import android.app.Activity;
- import android.view.Menu;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.Button;
- public class MainActivity extends Activity {
- Button start,pause,stop;
- @Override
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
- start=(Button)findViewById(R.id.button1);
- pause=(Button)findViewById(R.id.button2);
- stop=(Button)findViewById(R.id.button3);
- //creating media player
- final MediaPlayer mp=new MediaPlayer();
- try{
- //you can change the path, here path is external directory(e.g. sdcard) /Music/maine.mp3
- mp.setDataSource(Environment.getExternalStorageDirectory().getPath()+"/Music/maine.mp3");
- mp.prepare();
- }catch(Exception e){e.printStackTrace();}
- start.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View v) {
- mp.start();
- }
- });
- pause.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View v) {
- mp.pause();
- }
- });
- stop.setOnClickListener(new OnClickListener() {
- @Override
- public void onClick(View v) {
- mp.stop();
- }
- });
- }
- }
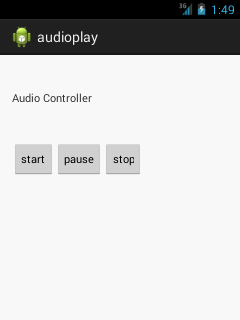
// button20 = (Button)findViewById(R.id.button20);
final MediaPlayer mp=MediaPlayer.create(this, R.raw.hello);//
//button20.setOnClickListener(new View.OnClickListener() {
@Override public void onClick(View View) { mp.start(); } }); //
///////////////////////////////////////
//////////////////////////////////////////////////
式碼中的extends和implements讓我感到很迷惑,現在終於弄明白它們之間的區別和用法了。
[c-sharp] view plain copy
//定義一個Runner介面
public inerface Runner
{
int ID = 1;
void run ();
}[java] view plain copy
//定義一個介面Animal,它繼承於父類介面Runner
interface Animal extends Runner
{
void breathe ();
}[c-sharp] view plain copy
//定義Fish類,它實現了Animal介面的方法run()和breather()
class Fish implements Animal
{
public void run () //實現了Animal方法run()
{
System.out.println(“fish is swimming”);
}
public void breather() //實現Animal的breather()方法
{
System.out.println(“fish is bubbing”);
}
}
//定義了一個抽象類LandAnimal,它實現了介面Animal的方法。
abstract LandAnimal implements Animal
{
public void breather ()
{
System.out.println(“LandAnimal is breathing”);
}
}
//定義了一個類Student,它繼承了類Person,並實現了Runner介面的方法run()。
class Student extends Person implements Runner
{
……
public void run ()
{
System.out.println(“the student is running”);
}
……
}
//定義了一個介面Flyer
interface Flyer
{
void fly ();
}
//定義了一個類Bird,它實現了Runner和Flyer這兩個介面定義的方法。
class Bird implements Runner , Flyer
{
public void run () //Runner介面定義的方法run()。
{
System.out.println(“the bird is running”);
}
public void fly () //Flyer介面定義的方法fly()。
{
System.out.println(“the bird is flying”);
}
}
//TestFish類
class TestFish
{
public static void main (String args[])
{
Fish f = new Fish();
int j = 0;
j = Runner.ID;
j = f.ID;
}
}